Adapting Adapter and building Bridge
Adapter and Bridge patterns are tow structural patterns described in GoF.
Adapter pattern basically converts one type of interface to another type of interface. Say you have an existing interface with the behaviors defined by you and your client is expecting another interface. Now how do you convert your existing interface into the interface your client expecting? Adapter pattern provides you the solution.
Bridge pattern decouple your abstraction and your implementation so that you can vary both your abstraction and implementation over time. Here implementation means designing a specific object with specific behavior and attributes, abstraction means abstracting the behaviors of the same said object. Without bridge the Abstraction and implementation are in same design hierarchy and bridge pattern separates the hierarchy of the two.
Example:
Suppose you have an interface called Pencil within which there is a method writeWithPencil() as:
public interface Pencil {
void writeWithPencil();
}
But your client has their interface Pen with the method write() as:
public interface Pen {
void write()
}
Now you want to give your client same transperncy without modifiying your existing interface. Actually you want to conert your Pencil into client's Pen. Here Pen is our target interface and Pencil is our adaptee.
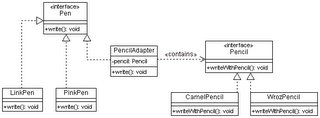
It's time to write our adapter:
public class PencilAdapter implements Pen {
private Pencil pencil;
public PencilAdapter(Pencil pencil) {
this.pencil = pencil;
}
public void write() {
pencil.writeWithPencil();
}
}
Now client code :
public class Main {
public static void main(String[] args) {
Pen p1 = new LinkPen();
Pen p2 = new PencilAdapter(new WrozPencil());
p1.write();
p2.write();
}
}
For bridge let us consider that we have Car interface as our abstraction and we are implementing different CarDriver. Without bridge we write
public class ConcreteCat implements CarDriver {
// code
}
It's not flexible as it the abstraction depends upon implementation via inheritance. But with bridge pattern we have our design like:
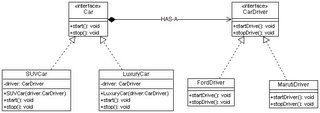
Client code:
public class Main() {
public static void main(String[] args) {
Car lCar = new LuxuryCar(new FordDrive());
lCar.start();
}
}
Adapter pattern basically converts one type of interface to another type of interface. Say you have an existing interface with the behaviors defined by you and your client is expecting another interface. Now how do you convert your existing interface into the interface your client expecting? Adapter pattern provides you the solution.
Bridge pattern decouple your abstraction and your implementation so that you can vary both your abstraction and implementation over time. Here implementation means designing a specific object with specific behavior and attributes, abstraction means abstracting the behaviors of the same said object. Without bridge the Abstraction and implementation are in same design hierarchy and bridge pattern separates the hierarchy of the two.
Example:
Suppose you have an interface called Pencil within which there is a method writeWithPencil() as:
public interface Pencil {
void writeWithPencil();
}
But your client has their interface Pen with the method write() as:
public interface Pen {
void write()
}
Now you want to give your client same transperncy without modifiying your existing interface. Actually you want to conert your Pencil into client's Pen. Here Pen is our target interface and Pencil is our adaptee.
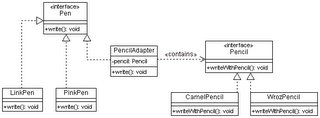
It's time to write our adapter:
public class PencilAdapter implements Pen {
private Pencil pencil;
public PencilAdapter(Pencil pencil) {
this.pencil = pencil;
}
public void write() {
pencil.writeWithPencil();
}
}
Now client code :
public class Main {
public static void main(String[] args) {
Pen p1 = new LinkPen();
Pen p2 = new PencilAdapter(new WrozPencil());
p1.write();
p2.write();
}
}
For bridge let us consider that we have Car interface as our abstraction and we are implementing different CarDriver. Without bridge we write
public class ConcreteCat implements CarDriver {
// code
}
It's not flexible as it the abstraction depends upon implementation via inheritance. But with bridge pattern we have our design like:
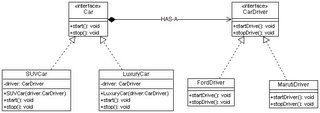
Client code:
public class Main() {
public static void main(String[] args) {
Car lCar = new LuxuryCar(new FordDrive());
lCar.start();
}
}
1 Comments:
Hi gautam,
Its very nice explanation about adapter and bridge patterns. Thanks!
Btw, which tool have you used for those UML diagrams?
Cheers!
By
Ananth, at 1:17 AM
Post a Comment
<< Home